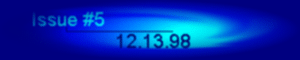


By MA SNART
Scripts...Scripts...Scripts...
Without a doubt nearly every Role-Playing Game you
have ever played has used Scripts. In fact most games made today use
scripts in some form or other.
So what do Scripts do? And how do you get QBasic to
use them? I hope to answer those questions, and even more, in this
series of articles. I'll even get into some more creative and advanced
things later on [like "compiling" a Script to run faster, and even
using a Script to program your program].
What are Scripts?
Well, for example, when you are programming a game
such as a RPG you become faced with several problems trying to
integrate all the things you want done into one program. Let's say in
your RPG you have 17 'worlds' each with several towns and in each town
you have several shops for items, weapons and whatnot. Because a single
.EXE program that contains every shop within every town in every
'world' would be too large to manage; you can break it apart into small
.EXEs for each shop, town and 'world'. This of course can be just as
unmanageable, because you end up repeating a lot of the core code you
really don't need to. The other way to go is to break that big program
apart and only leave in what you would have repeated in all the small
programs [graphics engine, sound engine, etc.] and add a new piece I'll
call a INTERPRETER. You then take all the details of each
room/shop/town/'world' and edit them into a script. This nice smaller
.EXE only has to OPEN a Script and INTERPRET it, done correctly it will
do the same as both the other larger EXE and the many smaller EXEs.
Scripts have been used for just about everything
from the Artificial Intelligence of the monsters in QUAKE to unit
abilities in CIVILIZATION II. But the easiest to understand and program
for are RPG cut-scenes and NPC dialog, so this is where we will begin.
How to get QBasic to use scripts?
Just imagine for a moment that you created a
programming language...Would it be like QBasic?...Or would you make
something along the lines of Assembler?...Would the syntax be quite
easy to learn?...Or would you need a 1,000 page manual to understand
it?
These are some pretty important questions as a
SCRIPT can be it's very own programming language. Your main program can
basically become an Operating System designed just to run your script
files. So before you read on take some time to decide just what you
want your SCRIPTS to do...And...Compare this to what you want your game
to do.
Okay, we'll begin with a simple SCRIPT format, that
can easily be expanded to meet future needs...[some may find what
follows very basic...I'm doing it this way just to get everybody up to
speed]
To start off you'll need to know what you want your
script to do. In this beginning article we only need it to tell the
game what the NPCs say.
*******What the script should tell the game: [version 1]
A] What a NPC says when 'talked' too by the player.
That's it for now, it is a
simple and good start, as we can build on it later. Okay first off we
will need a way to show the player what the NPC says. So in the main
EXE we will code a SUB I'll call MESSAGEBOX.
*******MESSAGEBOX will be
passed a STRING of text that it will write on the screen, then wait for
the player to press a key, before RETURNing.
Okay
now that we have a backbone for our Script to operate on, we will now
need a brain to read the script. Introducing the INTERPRETER.
The idea behind it is to READ in a Script file and
tell the EXE what the Script file wants done. To do this we need to
come up with Script language, by creating KEYWORDS and a SYNTAX. It
doesn't have to be fancy [yet!] so I'll obey the K.I.S.S. principal
[Keep It Simple Stupid]. We will use a KEYWORD followed by perimeters
system. So as to allow our Script language to expand we will allow our
KEYWORDS to have different STRING lengths as long as a colon follows
them [:].
So our first KEYWORD is 'MESSAGE BOX:'. Anything after it will be sent
as a text STRING to the MESSAGEBOX SUB. Simple enough.
Our simple version 1 INTERPRETER looks something like this:
[This is untested pseudo code...]
SUB SCRIPTINTERPRETER (SCRIPTFILENAME$)
OPEN SCRIPTFILENAME$ FOR INPUT AS #1
DO UNTIL EOF(1)
INPUT #1, SCRIPTCODE$
IF MID$(SCRIPTCODE$,1,1) <> "*" THEN
KEYWORD$=""
PERAMITER$=""
FOR I=1 to LEN(SCRIPTCODE$)
IF MID$(SCRIPTCODE$,I,1) = ":" THEN
KEYWORD$ = MID$(SCRIPTCODE$,1,I-1)
PERAMITER$ = MID$(SCRIPTCODE$,I+1,LEN(SCRIPTCODE$))
EXIT FOR
END IF
NEXT I
SELECT CASE KEYWORD$
CASE "MESSAGE BOX"
CALL MESSAGEBOX(PERAMITER$)
END SELECT
END IF
LOOP
CLOSE #1
RETURN
Okay now to explain
it...First off we open the Script file, then create a DO-UNTIL loop
that will continue until we have no more Script lines to read...We get
our first Scriptcode line, and see if the first Character is a astrik
["*"...this will allow you to enter comments]. If it isn't an astrik
then the line of code needs to be INTERPRETED. The next FOR-NEXT loop
checks the code letter by letter until it finds the KEYWORD separator
[a colon ":"]. All of the characters before the colon are entered into
the KEYWORD$ variable, everything after is then placed in the
PERAMITER$ variable...Then the REAL meat of the INTERPRETER begins, we
do a CASE select of KEYWORD$ to find a match. If it matches then the
correct subroutine is CALLed and sent the data in PERAMITER$.... This
can easily be modified by adding more CASE "KEYWORD" statements to the
SELECT-CASE portion of the Subroutine [If you want you can even allow
different KEYWORDS to mean the same thing by adding them to the CASE
statement...like: CASE "MESSAGE BOX","MB","M BOX" and so on...as long
as it doesn't contain a colon ":"].
To write the Scripts; you can use NOTEPAD, EDIT.COM
even QBASIC as long as it can be read as a TXT file your all set.
Although you can give it any extension you wish, for these articles,
I'll give the Scripts a .TXT extension.
Example Script [this should work with our current INTERPRETER]:
*This is the first script!
*The line below will allow us to do a MESSAGE BOX
MESSAGE BOX: What do you think?
*Okay now this maybe simple so far but check back for the
*next article installment
MESSAGE BOX: Bye-Bye!
Next time I'll add a bit more functions to the Script and show you how to do some real Cut-scenes.
Back to Top
This tutorial originally appeared in QBasic: The Magazine Issue 1.